PHObjectHandleVector< BASE > Class Template Reference
Base class for vectors of PHObject's handles. More...
#include <PHObjectHandleVector.h>
Inheritance diagram for PHObjectHandleVector< BASE >:
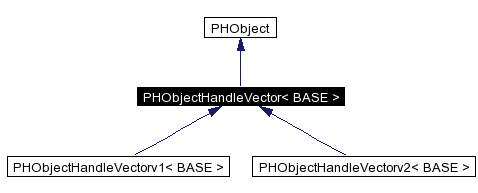
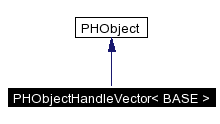
Public Types | |
typedef PHObjectHandle< BASE > | value_type |
typedef const value_type & | const_reference |
typedef value_type & | reference |
typedef unsigned int | size_type |
typedef PHObjectHandleVectorIterator< BASE > | iterator |
typedef PHObjectHandleVectorConstIterator< BASE > | const_iterator |
Public Member Functions | |
virtual | ~PHObjectHandleVector () |
virtual value_type & | add (const size_type &index)=0 |
Add a new object at position index and returns it. | |
virtual value_type & | add (const size_type &index, const value_type &value)=0 |
Add an existing object at position index. | |
iterator | begin () |
const_iterator | begin () const |
virtual size_type | capacity () const |
virtual PHObjectHandleVector< BASE > * | clone () const |
Virtual copy constructor. | |
virtual PHObjectHandleVector< BASE > * | create () const |
Virtual default constructor. | |
virtual bool | empty () const |
iterator | end () |
const_iterator | end () const |
virtual iterator | erase (iterator first, iterator last) |
virtual iterator | erase (iterator pos) |
virtual const_reference | get (const size_type &index) const |
virtual reference | get (const size_type &index) |
virtual void | identify (std::ostream &os=std::cout) const |
identify Function from PHObject | |
virtual iterator | insert (iterator pos, size_t n, const value_type &value) |
virtual iterator | insert (iterator pos, const value_type &value) |
virtual int | isValid () const |
isValid returns non zero if object contains vailid data | |
virtual bool | remove (const size_type &index) |
virtual void | reserve (const size_type &thesize) |
virtual void | Reset () |
Clear Event. | |
virtual bool | resize (const size_type &newsize, const value_type &value) |
virtual bool | resize (const size_type &newsize) |
virtual size_type | size () const |
Protected Member Functions | |
PHObjectHandleVector< BASE > & | operator= (const PHObjectHandleVector< BASE > &) |
Detailed Description
template<typename BASE>
class PHObjectHandleVector< BASE >
Base class for vectors of PHObject's handles.
Definition at line 312 of file PHObjectHandleVector.h.
Member Typedef Documentation
|
Definition at line 321 of file PHObjectHandleVector.h. Referenced by PHObjectHandleVector< BASE >::begin(), and PHObjectHandleVector< BASE >::end(). |
|
Definition at line 317 of file PHObjectHandleVector.h. Referenced by PHObjectHandleVector< BASE >::get(). |
|
Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 320 of file PHObjectHandleVector.h. Referenced by PHObjectHandleVector< BASE >::begin(), PHObjectHandleVector< BASE >::end(), PHObjectHandleVector< BASE >::erase(), and PHObjectHandleVector< BASE >::insert(). |
|
Definition at line 318 of file PHObjectHandleVector.h. Referenced by PHObjectHandleVector< BASE >::get(). |
|
Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 319 of file PHObjectHandleVector.h. Referenced by PHObjectHandleVector< BASE >::capacity(), PHObjectHandleVector< BASE >::get(), PHObjectHandleVector< BASE >::remove(), PHObjectHandleVector< BASE >::reserve(), PHObjectHandleVector< BASE >::resize(), and PHObjectHandleVector< BASE >::size(). |
|
Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 316 of file PHObjectHandleVector.h. Referenced by PHObjectHandleVector< BASE >::insert(), and PHObjectHandleVector< BASE >::resize(). |
Constructor & Destructor Documentation
|
Definition at line 323 of file PHObjectHandleVector.h. |
Member Function Documentation
|
Add an existing object at position index.
Implemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. |
|
Add a new object at position index and returns it.
Implemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. |
|
Definition at line 335 of file PHObjectHandleVector.h. References PHObjectHandleVector< BASE >::const_iterator. |
|
|
Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 338 of file PHObjectHandleVector.h. References PHObjectHandleVector< BASE >::size_type. |
|
Virtual copy constructor.
Reimplemented from PHObject. Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 341 of file PHObjectHandleVector.h. |
|
Virtual default constructor.
Reimplemented from PHObject. Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 344 of file PHObjectHandleVector.h. |
|
Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 347 of file PHObjectHandleVector.h. |
|
Definition at line 353 of file PHObjectHandleVector.h. References PHObjectHandleVector< BASE >::const_iterator, and PHObjectHandleVector< BASE >::size(). |
|
Definition at line 350 of file PHObjectHandleVector.h. References PHObjectHandleVector< BASE >::iterator, and PHObjectHandleVector< BASE >::size(). Referenced by PHObjectHandleVectorv2< BASE, DERIVED >::insert(), PHObjectHandleVectorv1< BASE, DERIVED >::insert(), and PHObjectHandleVectorv2< BASE, DERIVED >::resize(). |
|
Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 359 of file PHObjectHandleVector.h. References PHObjectHandleVector< BASE >::iterator. |
|
Definition at line 356 of file PHObjectHandleVector.h. References PHObjectHandleVector< BASE >::iterator. |
|
Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 365 of file PHObjectHandleVector.h. References PHObjectHandleVector< BASE >::reference, and PHObjectHandleVector< BASE >::size_type. |
|
Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 362 of file PHObjectHandleVector.h. References PHObjectHandleVector< BASE >::const_reference, and PHObjectHandleVector< BASE >::size_type. Referenced by PHObjectHandleVectorConstIterator< BASE >::operator *(), and PHObjectHandleVectorIterator< BASE >::operator *(). |
|
identify Function from PHObject
Reimplemented from PHObject. Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 368 of file PHObjectHandleVector.h. |
|
Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 374 of file PHObjectHandleVector.h. References PHObjectHandleVector< BASE >::iterator, and PHObjectHandleVector< BASE >::value_type. |
|
Definition at line 371 of file PHObjectHandleVector.h. References PHObjectHandleVector< BASE >::iterator, and PHObjectHandleVector< BASE >::value_type. |
|
isValid returns non zero if object contains vailid data
Reimplemented from PHObject. Definition at line 377 of file PHObjectHandleVector.h. |
|
|
|
Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 380 of file PHObjectHandleVector.h. References PHObjectHandleVector< BASE >::size_type. |
|
Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 383 of file PHObjectHandleVector.h. References PHObjectHandleVector< BASE >::size_type. |
|
Clear Event.
Reimplemented from PHObject. Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 386 of file PHObjectHandleVector.h. |
|
Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 392 of file PHObjectHandleVector.h. References PHObjectHandleVector< BASE >::size_type. |
|
Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 389 of file PHObjectHandleVector.h. References PHObjectHandleVector< BASE >::size_type, and PHObjectHandleVector< BASE >::value_type. |
|
Reimplemented in PHObjectHandleVectorv1< BASE, DERIVED >, and PHObjectHandleVectorv2< BASE, DERIVED >. Definition at line 395 of file PHObjectHandleVector.h. References PHObjectHandleVector< BASE >::size_type. Referenced by PHObjectHandleVector< BASE >::end(). |
The documentation for this class was generated from the following file: