PHObjectHandleVectorv2< BASE, DERIVED > Class Template Reference
Implementation of PHObjectHandleVector. More...
#include <PHObjectHandleVectorv2.h>
Inheritance diagram for PHObjectHandleVectorv2< BASE, DERIVED >:
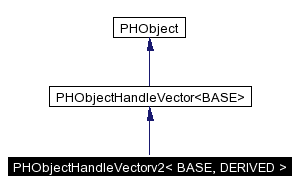
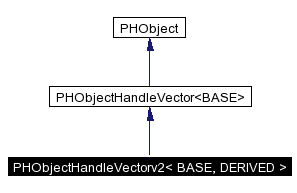
Public Types | |
typedef PHObjectHandleVectorv2< BASE, DERIVED > | self |
typedef PHObjectHandle< BASE > | value_type |
typedef unsigned int | size_type |
typedef PHObjectHandleVectorIterator< BASE > | iterator |
Public Member Functions | |
PHObjectHandleVectorv2 () | |
PHObjectHandleVectorv2 (const self &other) | |
self & | operator= (const self &other) |
virtual | ~PHObjectHandleVectorv2 () |
virtual value_type | add (const size_type &index) |
Add a new object at position index and returns it. | |
virtual value_type | add (const size_type &index, const value_type &value) |
Add an existing object at position index. | |
virtual size_type | capacity () const |
virtual self * | clone () const |
Virtual copy constructor. | |
virtual self * | create () const |
Virtual default constructor. | |
virtual bool | empty () const |
virtual iterator | erase (iterator first, iterator last) |
virtual iterator | erase (iterator pos) |
virtual const value_type & | get (const size_type &index) const |
virtual value_type & | get (const size_type &index) |
virtual void | identify (std::ostream &os=std::cout) const |
identify Function from PHObject | |
virtual iterator | insert (iterator pos, size_type n, const value_type &value) |
virtual iterator | insert (iterator pos, const value_type &value) |
virtual bool | remove (const size_type &index) |
virtual void | reserve (const size_type &thesize) |
virtual void | Reset () |
Clear Event. | |
virtual bool | resize (const size_type &newsize, const value_type &value) |
virtual bool | resize (const size_type &newsize) |
virtual size_type | size () const |
Detailed Description
template<typename BASE, typename DERIVED>
class PHObjectHandleVectorv2< BASE, DERIVED >
Implementation of PHObjectHandleVector.
Using std::vector as underlying technology. Requirements on type BASE and DERIVED: must have a BASE* create() method (aka virtual ctor) must have a BASE* clone() method (aka virtual copy ctor) must have an << operator
Definition at line 17 of file PHObjectHandleVectorv2.h.
Member Typedef Documentation
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 24 of file PHObjectHandleVectorv2.h. Referenced by PHObjectHandleVectorv2< BASE, DERIVED >::erase(), PHObjectHandleVectorv2< BASE, DERIVED >::insert(), and PHObjectHandleVectorv2< BASE, DERIVED >::resize(). |
|
Definition at line 21 of file PHObjectHandleVectorv2.h. |
|
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 22 of file PHObjectHandleVectorv2.h. Referenced by PHObjectHandleVectorv2< BASE, DERIVED >::add(), PHObjectHandleVectorv2< BASE, DERIVED >::get(), PHObjectHandleVectorv2< BASE, DERIVED >::insert(), and PHObjectHandleVectorv2< BASE, DERIVED >::resize(). |
Constructor & Destructor Documentation
|
Definition at line 111 of file PHObjectHandleVectorv2.h. |
|
|
|
Definition at line 151 of file PHObjectHandleVectorv2.h. |
Member Function Documentation
|
Add an existing object at position index.
Implements PHObjectHandleVector< BASE >. Definition at line 185 of file PHObjectHandleVectorv2.h. References PHObjectHandleVectorv2< BASE, DERIVED >::capacity(), PHObjectHandleVectorv2< BASE, DERIVED >::get(), PHObjectHandle< BASE >::get(), PHObjectHandleVectorv2< BASE, DERIVED >::size_type, and PHObjectHandleVectorv2< BASE, DERIVED >::value_type. |
|
Add a new object at position index and returns it.
Implements PHObjectHandleVector< BASE >. Definition at line 159 of file PHObjectHandleVectorv2.h. References PHObjectHandleVectorv2< BASE, DERIVED >::capacity(), PHObjectHandleVectorv2< BASE, DERIVED >::get(), PHObjectHandleVectorv2< BASE, DERIVED >::size_type, and PHObjectHandleVectorv2< BASE, DERIVED >::value_type. |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 228 of file PHObjectHandleVectorv2.h. Referenced by PHObjectHandleVectorv2< BASE, DERIVED >::add(), PHObjectHandleVectorv2< BASE, DERIVED >::identify(), PHObjectHandleVectorv2< BASE, DERIVED >::insert(), and PHObjectHandleVectorv2< BASE, DERIVED >::Reset(). |
|
Virtual copy constructor.
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 254 of file PHObjectHandleVectorv2.h. |
|
Virtual default constructor.
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 262 of file PHObjectHandleVectorv2.h. |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 42 of file PHObjectHandleVectorv2.h. |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 46 of file PHObjectHandleVectorv2.h. References PHObjectHandleVectorv2< BASE, DERIVED >::erase(), and PHObjectHandleVectorv2< BASE, DERIVED >::iterator. |
|
Definition at line 311 of file PHObjectHandleVectorv2.h. References PHObjectHandleVectorv2< BASE, DERIVED >::iterator. Referenced by PHObjectHandleVectorv2< BASE, DERIVED >::erase(), PHObjectHandleVectorv2< BASE, DERIVED >::remove(), and PHObjectHandleVectorv2< BASE, DERIVED >::resize(). |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 378 of file PHObjectHandleVectorv2.h. References BASE, PHObjectHandleVectorv2< BASE, DERIVED >::size_type, and PHObjectHandleVectorv2< BASE, DERIVED >::value_type. |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 363 of file PHObjectHandleVectorv2.h. References BASE, PHObjectHandleVectorv2< BASE, DERIVED >::size_type, and PHObjectHandleVectorv2< BASE, DERIVED >::value_type. Referenced by PHObjectHandleVectorv2< BASE, DERIVED >::add(). |
|
identify Function from PHObject
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 393 of file PHObjectHandleVectorv2.h. References PHObjectHandleVectorv2< BASE, DERIVED >::capacity(), and PHObjectHandleVectorv2< BASE, DERIVED >::size(). |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 56 of file PHObjectHandleVectorv2.h. References PHObjectHandleVectorv2< BASE, DERIVED >::insert(), PHObjectHandleVectorv2< BASE, DERIVED >::iterator, and PHObjectHandleVectorv2< BASE, DERIVED >::value_type. |
|
|
|
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 467 of file PHObjectHandleVectorv2.h. References PHObjectHandleVector< BASE >::begin(), PHObjectHandleVectorv2< BASE, DERIVED >::erase(), PHObjectHandleVectorv2< BASE, DERIVED >::size(), and PHObjectHandleVectorv2< BASE, DERIVED >::size_type. |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 486 of file PHObjectHandleVectorv2.h. References PHObjectHandleVectorv2< BASE, DERIVED >::size_type. |
|
Clear Event.
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 498 of file PHObjectHandleVectorv2.h. References PHObjectHandleVectorv2< BASE, DERIVED >::capacity(). |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 67 of file PHObjectHandleVectorv2.h. References PHObjectHandleVectorv2< BASE, DERIVED >::resize(), PHObjectHandleVectorv2< BASE, DERIVED >::size_type, and PHObjectHandleVectorv2< BASE, DERIVED >::value_type. |
|
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 575 of file PHObjectHandleVectorv2.h. Referenced by PHObjectHandleVectorv2< BASE, DERIVED >::identify(), PHObjectHandleVectorv2< BASE, DERIVED >::remove(), and PHObjectHandleVectorv2< BASE, DERIVED >::resize(). |
The documentation for this class was generated from the following file: