PHObjectHandleVectorIterator< BASE > Class Template Reference
PHObjectHandleVector iterator (non const version). More...
#include <PHObjectHandleVector.h>
Collaboration diagram for PHObjectHandleVectorIterator< BASE >:
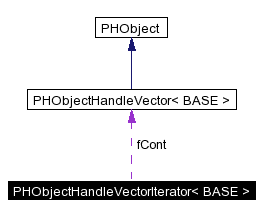
Detailed Description
template<typename BASE>
class PHObjectHandleVectorIterator< BASE >
PHObjectHandleVector iterator (non const version).
Definition at line 18 of file PHObjectHandleVector.h.
Member Typedef Documentation
|
Definition at line 28 of file PHObjectHandleVector.h. |
|
Definition at line 25 of file PHObjectHandleVector.h. Referenced by PHObjectHandleVectorIterator< BASE >::PHObjectHandleVectorIterator(). |
|
|
|
Definition at line 23 of file PHObjectHandleVector.h. |
|
Definition at line 29 of file PHObjectHandleVector.h. |
|
Definition at line 27 of file PHObjectHandleVector.h. |
|
Definition at line 24 of file PHObjectHandleVector.h. |
Constructor & Destructor Documentation
|
Definition at line 31 of file PHObjectHandleVector.h. |
|
Definition at line 33 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::container. |
|
Definition at line 38 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::fCont, PHObjectHandleVectorIterator< BASE >::fPos, and PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 44 of file PHObjectHandleVector.h. |
Member Function Documentation
|
Definition at line 49 of file PHObjectHandleVector.h. Referenced by PHObjectHandleVectorIterator< BASE >::operator--(). |
|
Definition at line 48 of file PHObjectHandleVector.h. Referenced by PHObjectHandleVectorIterator< BASE >::operator++(). |
|
Definition at line 415 of file PHObjectHandleVector.h. References PHObjectHandleVector< BASE >::get(). |
|
Definition at line 100 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::fCont, PHObjectHandleVectorIterator< BASE >::fPos, and PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 112 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::fPos, and PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 77 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::difference_type, and PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 55 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::increment(), and PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 51 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::increment(), and PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 73 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::difference_type, and PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 106 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::fPos, and PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 88 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::difference_type, and PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 66 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::decrement(), and PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 62 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::decrement(), and PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 84 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::difference_type, and PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 123 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 128 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 95 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::fCont, PHObjectHandleVectorIterator< BASE >::fPos, and PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 133 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 138 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::iterator. |
|
Definition at line 118 of file PHObjectHandleVector.h. References PHObjectHandleVectorIterator< BASE >::difference_type, and PHObjectHandleVectorIterator< BASE >::iterator. |
The documentation for this class was generated from the following file: