PHObjectHandleVectorv1< BASE, DERIVED > Class Template Reference
Implementation of PHObjectHandleVector. More...
#include <PHObjectHandleVectorv1.h>
Inheritance diagram for PHObjectHandleVectorv1< BASE, DERIVED >:
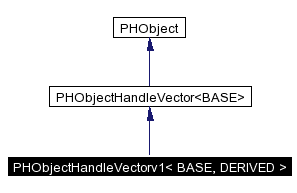
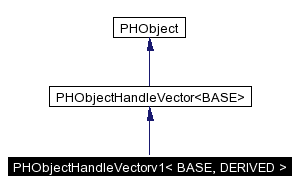
Public Types | |
typedef PHObjectHandleVectorv1< BASE, DERIVED > | self |
typedef PHObjectHandle< BASE > | value_type |
typedef unsigned int | size_type |
typedef PHObjectHandleVectorIterator< BASE > | iterator |
Public Member Functions | |
PHObjectHandleVectorv1 () | |
PHObjectHandleVectorv1 (const self &other) | |
self & | operator= (const self &other) |
virtual | ~PHObjectHandleVectorv1 () |
virtual value_type & | add (const size_type &index) |
Add a new object at position index and returns it. | |
virtual value_type & | add (const size_type &index, const value_type &value) |
Add an existing object at position index. | |
virtual size_type | capacity () const |
virtual self * | clone () const |
Virtual copy constructor. | |
virtual self * | create () const |
Virtual default constructor. | |
virtual bool | empty () const |
virtual iterator | erase (iterator first, iterator last) |
virtual iterator | erase (iterator pos) |
virtual const value_type & | get (const size_type &index) const |
virtual value_type & | get (const size_type &index) |
virtual void | identify (std::ostream &os=std::cout) const |
identify Function from PHObject | |
virtual iterator | insert (iterator pos, size_type n, const value_type &value) |
virtual iterator | insert (iterator pos, const value_type &value) |
virtual bool | remove (const size_type &index) |
virtual void | reserve (const size_type &thesize) |
virtual void | Reset () |
Clear Event. | |
virtual bool | resize (const size_type &newsize, const value_type &value) |
virtual bool | resize (const size_type &newsize) |
virtual size_type | size () const |
Detailed Description
template<typename BASE, typename DERIVED>
class PHObjectHandleVectorv1< BASE, DERIVED >
Implementation of PHObjectHandleVector.
Using TClonesArray as underlying technology. Requirements on type BASE and DERIVED: must have a BASE* create() method (aka virtual ctor) must have a BASE* clone() method (aka virtual copy ctor) must have an << operator
Definition at line 18 of file PHObjectHandleVectorv1.h.
Member Typedef Documentation
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 25 of file PHObjectHandleVectorv1.h. Referenced by PHObjectHandleVectorv1< BASE, DERIVED >::erase(), and PHObjectHandleVectorv1< BASE, DERIVED >::insert(). |
|
Definition at line 22 of file PHObjectHandleVectorv1.h. |
|
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 23 of file PHObjectHandleVectorv1.h. Referenced by PHObjectHandleVectorv1< BASE, DERIVED >::add(), PHObjectHandleVectorv1< BASE, DERIVED >::get(), PHObjectHandleVectorv1< BASE, DERIVED >::identify(), PHObjectHandleVectorv1< BASE, DERIVED >::insert(), and PHObjectHandleVectorv1< BASE, DERIVED >::resize(). |
Constructor & Destructor Documentation
|
Definition at line 99 of file PHObjectHandleVectorv1.h. |
|
|
|
Definition at line 136 of file PHObjectHandleVectorv1.h. |
Member Function Documentation
|
Add an existing object at position index.
Implements PHObjectHandleVector< BASE >. Definition at line 169 of file PHObjectHandleVectorv1.h. References PHObjectHandleVectorv1< BASE, DERIVED >::capacity(), PHObjectHandleVectorv1< BASE, DERIVED >::get(), PHObjectHandle< BASE >::get(), PHObjectHandleVectorv1< BASE, DERIVED >::size_type, and PHObjectHandleVectorv1< BASE, DERIVED >::value_type. |
|
Add a new object at position index and returns it.
Implements PHObjectHandleVector< BASE >. Definition at line 144 of file PHObjectHandleVectorv1.h. References PHObjectHandleVectorv1< BASE, DERIVED >::capacity(), PHObjectHandleVectorv1< BASE, DERIVED >::get(), PHObjectHandleVectorv1< BASE, DERIVED >::size_type, and PHObjectHandleVectorv1< BASE, DERIVED >::value_type. |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 208 of file PHObjectHandleVectorv1.h. Referenced by PHObjectHandleVectorv1< BASE, DERIVED >::add(), PHObjectHandleVectorv1< BASE, DERIVED >::identify(), and PHObjectHandleVectorv1< BASE, DERIVED >::insert(). |
|
Virtual copy constructor.
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 251 of file PHObjectHandleVectorv1.h. |
|
Virtual default constructor.
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 259 of file PHObjectHandleVectorv1.h. |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 43 of file PHObjectHandleVectorv1.h. |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 47 of file PHObjectHandleVectorv1.h. References PHObjectHandleVectorv1< BASE, DERIVED >::erase(), and PHObjectHandleVectorv1< BASE, DERIVED >::iterator. |
|
Definition at line 296 of file PHObjectHandleVectorv1.h. References PHObjectHandleVectorv1< BASE, DERIVED >::iterator. Referenced by PHObjectHandleVectorv1< BASE, DERIVED >::erase(), and PHObjectHandleVectorv1< BASE, DERIVED >::remove(). |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 341 of file PHObjectHandleVectorv1.h. References PHObjectHandleVectorv1< BASE, DERIVED >::size_type, and PHObjectHandleVectorv1< BASE, DERIVED >::value_type. |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 330 of file PHObjectHandleVectorv1.h. References PHObjectHandleVectorv1< BASE, DERIVED >::size_type, and PHObjectHandleVectorv1< BASE, DERIVED >::value_type. Referenced by PHObjectHandleVectorv1< BASE, DERIVED >::add(). |
|
identify Function from PHObject
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 352 of file PHObjectHandleVectorv1.h. References PHObjectHandleVectorv1< BASE, DERIVED >::capacity(), PHObjectHandleVectorv1< BASE, DERIVED >::size(), and PHObjectHandleVectorv1< BASE, DERIVED >::value_type. |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 57 of file PHObjectHandleVectorv1.h. References PHObjectHandleVectorv1< BASE, DERIVED >::insert(), PHObjectHandleVectorv1< BASE, DERIVED >::iterator, and PHObjectHandleVectorv1< BASE, DERIVED >::value_type. |
|
|
|
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 416 of file PHObjectHandleVectorv1.h. References PHObjectHandleVector< BASE >::begin(), PHObjectHandleVectorv1< BASE, DERIVED >::erase(), PHObjectHandleVectorv1< BASE, DERIVED >::size(), and PHObjectHandleVectorv1< BASE, DERIVED >::size_type. |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 432 of file PHObjectHandleVectorv1.h. References PHObjectHandleVectorv1< BASE, DERIVED >::size_type. Referenced by PHObjectHandleVectorv1< BASE, DERIVED >::resize(). |
|
Clear Event.
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 448 of file PHObjectHandleVectorv1.h. |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 68 of file PHObjectHandleVectorv1.h. References PHObjectHandleVectorv1< BASE, DERIVED >::resize(), PHObjectHandleVectorv1< BASE, DERIVED >::size_type, and PHObjectHandleVectorv1< BASE, DERIVED >::value_type. |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 458 of file PHObjectHandleVectorv1.h. References PHObjectHandleVectorv1< BASE, DERIVED >::reserve(), PHObjectHandleVectorv1< BASE, DERIVED >::size_type, and PHObjectHandleVectorv1< BASE, DERIVED >::value_type. Referenced by PHObjectHandleVectorv1< BASE, DERIVED >::resize(). |
|
Reimplemented from PHObjectHandleVector< BASE >. Definition at line 490 of file PHObjectHandleVectorv1.h. Referenced by PHObjectHandleVectorv1< BASE, DERIVED >::identify(), and PHObjectHandleVectorv1< BASE, DERIVED >::remove(). |
The documentation for this class was generated from the following file: