PHObjectVectorSTL< BASE, DERIVED > Class Template Reference
Implementation of PHObjectVector. More...
#include <PHObjectVectorSTL.h>
Inheritance diagram for PHObjectVectorSTL< BASE, DERIVED >:
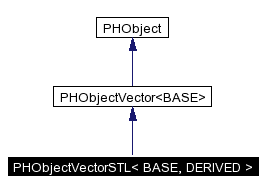
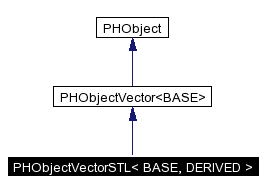
Public Types | |
typedef PHObjectVectorSTL< BASE, DERIVED > | self |
typedef BASE * | value_type |
typedef unsigned int | size_type |
Public Member Functions | |
PHObjectVectorSTL (const size_type defaultcapacity=10, const size_type maxcapacity=100) | |
PHObjectVectorSTL (const self &other) | |
self & | operator= (const self &other) |
virtual | ~PHObjectVectorSTL () |
virtual value_type | add (const size_type &index) |
Add a new object at position index, and returns it. | |
virtual value_type | add (const size_type &index, const value_type &value) |
Add an existing object at position index, and returns it. | |
virtual size_type | capacity () const |
Maximum size. | |
virtual PHObjectVectorSTL< BASE, DERIVED > * | clone () const |
Virtual copy constructor. | |
virtual PHObjectVectorSTL< BASE, DERIVED > * | create () const |
Virtual default constructor. | |
virtual bool | empty () const |
Whether this vector is empty. | |
virtual void | erase (const size_type &index) |
Erase one element. | |
virtual value_type | get (const size_type &index) const |
Get the index-th element. | |
virtual void | identify (std::ostream &os=std::cout) const |
Identify from PHObject. | |
virtual int | isValid () const |
isValid from PHObject. | |
virtual void | Reset () |
Reset from PHObject. | |
virtual bool | reserve (const size_type &) |
Request allocation for at least n elements. | |
virtual bool | resize (const size_type &newsize, value_type v=value_type()) |
Change the size. | |
virtual size_type | size () const |
Get the size of this vector. |
Detailed Description
template<typename BASE, typename DERIVED>
class PHObjectVectorSTL< BASE, DERIVED >
Implementation of PHObjectVector.
Using std::vector as underlying technology.
Definition at line 12 of file PHObjectVectorSTL.h.
Member Typedef Documentation
|
Definition at line 15 of file PHObjectVectorSTL.h. |
|
|
Reimplemented from PHObjectVector< BASE >. Definition at line 16 of file PHObjectVectorSTL.h. Referenced by PHObjectVectorSTL< BASE, DERIVED >::add(), and PHObjectVectorSTL< BASE, DERIVED >::resize(). |
Constructor & Destructor Documentation
|
Definition at line 96 of file PHObjectVectorSTL.h. References PHObjectVectorSTL< BASE, DERIVED >::size_type. |
|
|
|
Definition at line 145 of file PHObjectVectorSTL.h. |
Member Function Documentation
|
Add an existing object at position index, and returns it.
Implements PHObjectVector< BASE >. Definition at line 179 of file PHObjectVectorSTL.h. References PHObjectVectorSTL< BASE, DERIVED >::capacity(), PHObjectVectorSTL< BASE, DERIVED >::get(), PHObjectVectorSTL< BASE, DERIVED >::size_type, and PHObjectVectorSTL< BASE, DERIVED >::value_type. |
|
Add a new object at position index, and returns it.
Implements PHObjectVector< BASE >. Definition at line 153 of file PHObjectVectorSTL.h. References PHObjectVectorSTL< BASE, DERIVED >::capacity(), PHObjectVectorSTL< BASE, DERIVED >::get(), PHObjectVectorSTL< BASE, DERIVED >::size_type, and PHObjectVectorSTL< BASE, DERIVED >::value_type. |
|
Maximum size.
Implements PHObjectVector< BASE >. Definition at line 220 of file PHObjectVectorSTL.h. Referenced by PHObjectVectorSTL< BASE, DERIVED >::add(), and PHObjectVectorSTL< BASE, DERIVED >::identify(). |
|
Virtual copy constructor.
Implements PHObjectVector< BASE >. Definition at line 248 of file PHObjectVectorSTL.h. |
|
Virtual default constructor.
Implements PHObjectVector< BASE >. Definition at line 256 of file PHObjectVectorSTL.h. |
|
Whether this vector is empty.
Reimplemented from PHObjectVector< BASE >. Definition at line 42 of file PHObjectVectorSTL.h. |
|
Erase one element.
Implements PHObjectVector< BASE >. Definition at line 377 of file PHObjectVectorSTL.h. References PHObjectVectorSTL< BASE, DERIVED >::size_type. |
|
Get the index-th element.
Implements PHObjectVector< BASE >. Definition at line 303 of file PHObjectVectorSTL.h. References PHObjectVectorSTL< BASE, DERIVED >::size_type. Referenced by PHObjectVectorSTL< BASE, DERIVED >::add(), and PHObjectVectorSTL< BASE, DERIVED >::identify(). |
|
Identify from PHObject.
Implements PHObjectVector< BASE >. Definition at line 311 of file PHObjectVectorSTL.h. References BASE, PHObjectVectorSTL< BASE, DERIVED >::capacity(), PHObjectVectorSTL< BASE, DERIVED >::get(), and PHObjectVectorSTL< BASE, DERIVED >::size(). |
|
isValid from PHObject.
Implements PHObjectVector< BASE >. Definition at line 54 of file PHObjectVectorSTL.h. |
|
|
|
Request allocation for at least n elements.
Implements PHObjectVector< BASE >. Definition at line 345 of file PHObjectVectorSTL.h. References PHObjectVectorSTL< BASE, DERIVED >::size_type. |
|
Reset from PHObject.
Implements PHObjectVector< BASE >. Definition at line 364 of file PHObjectVectorSTL.h. References PHObjectVectorSTL< BASE, DERIVED >::size_type. |
|
Change the size.
Implements PHObjectVector< BASE >. Definition at line 387 of file PHObjectVectorSTL.h. References PHObjectVectorSTL< BASE, DERIVED >::size(), PHObjectVectorSTL< BASE, DERIVED >::size_type, and PHObjectVectorSTL< BASE, DERIVED >::value_type. |
|
Get the size of this vector.
Implements PHObjectVector< BASE >. Definition at line 444 of file PHObjectVectorSTL.h. Referenced by PHObjectVectorSTL< BASE, DERIVED >::identify(), and PHObjectVectorSTL< BASE, DERIVED >::resize(). |
The documentation for this class was generated from the following file: