PHObjectVector< BASE > Class Template Reference
Base class for vectors of PHObject pointers. More...
#include <PHObjectVector.h>
Inheritance diagram for PHObjectVector< BASE >:
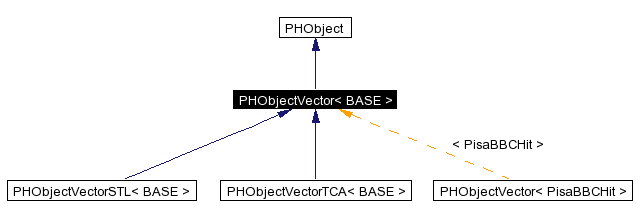
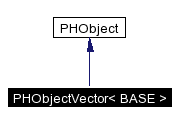
Public Types | |
typedef BASE * | value_type |
typedef const value_type & | const_reference |
typedef value_type & | reference |
typedef unsigned int | size_type |
Public Member Functions | |
virtual | ~PHObjectVector () |
virtual value_type | add (const size_type &index)=0 |
Inserts a new object at position index, and returns it. | |
virtual value_type | add (const size_type &index, const value_type &value)=0 |
Inserts an existing object at position index, and returns it. | |
virtual value_type | back () const |
Returns last element. | |
virtual size_type | capacity () const =0 |
Maximum size. | |
virtual PHObjectVector< BASE > * | clone () const =0 |
Virtual copy constructor. | |
virtual PHObjectVector< BASE > * | create () const =0 |
Virtual default constructor. | |
virtual bool | empty () const |
Whether this vector is empty. | |
virtual void | erase (const size_type &i)=0 |
Removes i-th element. | |
virtual value_type | front () const |
Returns first element. | |
virtual value_type | get (const size_type &index) const =0 |
Gets the index-th element. | |
virtual void | identify (std::ostream &os=std::cout) const =0 |
Identify from PHObject. | |
virtual int | isValid () const =0 |
isValid from PHObject. | |
virtual void | pop_back () |
Removes last element. | |
virtual void | push_back (const value_type &val) |
Inserts a new element at the end. | |
virtual void | Reset ()=0 |
Reset from PHObject. | |
virtual bool | reserve (const size_type &n)=0 |
Request allocation for at least n elements. | |
virtual bool | resize (const size_type &newsize, value_type v=value_type())=0 |
Change the size (either add or remove elements so that size() after this call is equal to newsize. | |
virtual size_type | size () const =0 |
Get the size of this vector. |
Detailed Description
template<class BASE>
class PHObjectVector< BASE >
Base class for vectors of PHObject pointers.
Definition at line 10 of file PHObjectVector.h.
Member Typedef Documentation
|
Reimplemented in PHObjectVectorTCA< BASE, DERIVED >. Definition at line 15 of file PHObjectVector.h. |
|
Reimplemented in PHObjectVectorTCA< BASE, DERIVED >. Definition at line 16 of file PHObjectVector.h. |
|
Reimplemented in PHObjectVectorSTL< BASE, DERIVED >, and PHObjectVectorTCA< BASE, DERIVED >. Definition at line 17 of file PHObjectVector.h. |
|
Reimplemented in PHObjectVectorSTL< BASE, DERIVED >, and PHObjectVectorTCA< BASE, DERIVED >. Definition at line 14 of file PHObjectVector.h. Referenced by PHObjectVector< PisaBBCHit >::back(). |
Constructor & Destructor Documentation
|
Definition at line 19 of file PHObjectVector.h. |
Member Function Documentation
|
Inserts an existing object at position index, and returns it.
Implemented in PHObjectVectorSTL< BASE, DERIVED >, and PHObjectVectorTCA< BASE, DERIVED >. |
|
Inserts a new object at position index, and returns it.
Implemented in PHObjectVectorSTL< BASE, DERIVED >, and PHObjectVectorTCA< BASE, DERIVED >. Referenced by PHObjectVector< PisaBBCHit >::push_back(), and PisaBBC::stepManager(). |
|
Returns last element.
Definition at line 28 of file PHObjectVector.h. Referenced by PisaBBC::stepManager(). |
|
Maximum size.
Implemented in PHObjectVectorSTL< BASE, DERIVED >, and PHObjectVectorTCA< BASE, DERIVED >. |
|
Virtual copy constructor.
Reimplemented from PHObject. Implemented in PHObjectVectorSTL< BASE, DERIVED >, and PHObjectVectorTCA< BASE, DERIVED >. |
|
Virtual default constructor.
Reimplemented from PHObject. Implemented in PHObjectVectorSTL< BASE, DERIVED >, and PHObjectVectorTCA< BASE, DERIVED >. |
|
Whether this vector is empty.
Reimplemented in PHObjectVectorSTL< BASE, DERIVED >, and PHObjectVectorTCA< BASE, DERIVED >. Definition at line 50 of file PHObjectVector.h. Referenced by PHObjectVector< PisaBBCHit >::back(), and PHObjectVector< PisaBBCHit >::pop_back(). |
|
Removes i-th element.
Implemented in PHObjectVectorSTL< BASE, DERIVED >, and PHObjectVectorTCA< BASE, DERIVED >. Referenced by PHObjectVector< PisaBBCHit >::pop_back(). |
|
Returns first element.
Definition at line 56 of file PHObjectVector.h. |
|
Gets the index-th element.
Implemented in PHObjectVectorSTL< BASE, DERIVED >, and PHObjectVectorTCA< BASE, DERIVED >. Referenced by PHObjectVector< PisaBBCHit >::back(), and PHObjectVector< PisaBBCHit >::front(). |
|
Identify from PHObject.
Reimplemented from PHObject. Implemented in PHObjectVectorSTL< BASE, DERIVED >, and PHObjectVectorTCA< BASE, DERIVED >. |
|
isValid from PHObject.
Reimplemented from PHObject. Implemented in PHObjectVectorSTL< BASE, DERIVED >, and PHObjectVectorTCA< BASE, DERIVED >. |
|
Removes last element.
Definition at line 68 of file PHObjectVector.h. |
|
Inserts a new element at the end.
Definition at line 77 of file PHObjectVector.h. |
|
Request allocation for at least n elements.
Implemented in PHObjectVectorSTL< BASE, DERIVED >, and PHObjectVectorTCA< BASE, DERIVED >. |
|
Reset from PHObject.
Reimplemented from PHObject. Implemented in PHObjectVectorSTL< BASE, DERIVED >, and PHObjectVectorTCA< BASE, DERIVED >. |
|
Change the size (either add or remove elements so that size() after this call is equal to newsize.
Implemented in PHObjectVectorSTL< BASE, DERIVED >, and PHObjectVectorTCA< BASE, DERIVED >. |
|
Get the size of this vector.
Implemented in PHObjectVectorSTL< BASE, DERIVED >, and PHObjectVectorTCA< BASE, DERIVED >. Referenced by PHObjectVector< PisaBBCHit >::back(), PHObjectVector< PisaBBCHit >::empty(), PisaBBC::finishEvent(), PHObjectVector< PisaBBCHit >::pop_back(), PHObjectVector< PisaBBCHit >::push_back(), and PisaBBC::stepManager(). |
The documentation for this class was generated from the following file: